Proof of address
Comprehensive capture and extraction of Proofs of address
There are multiple documents associated with a proof of address. Our API is able to distinguish them and to extract their information accordingly. Despite its status of non-official document, the proof of address remains a major document in the processes of confirming an individual's address and mitigating fraud.
Introduction
Datakeen's OCR service automatically extracts information from proof of address documents. The platform handles invoices from multiple public service providers, including water, gas, energy and internet providers.
- Information is captured as structured data and can be extracted as a .json file through our API.
Water bill
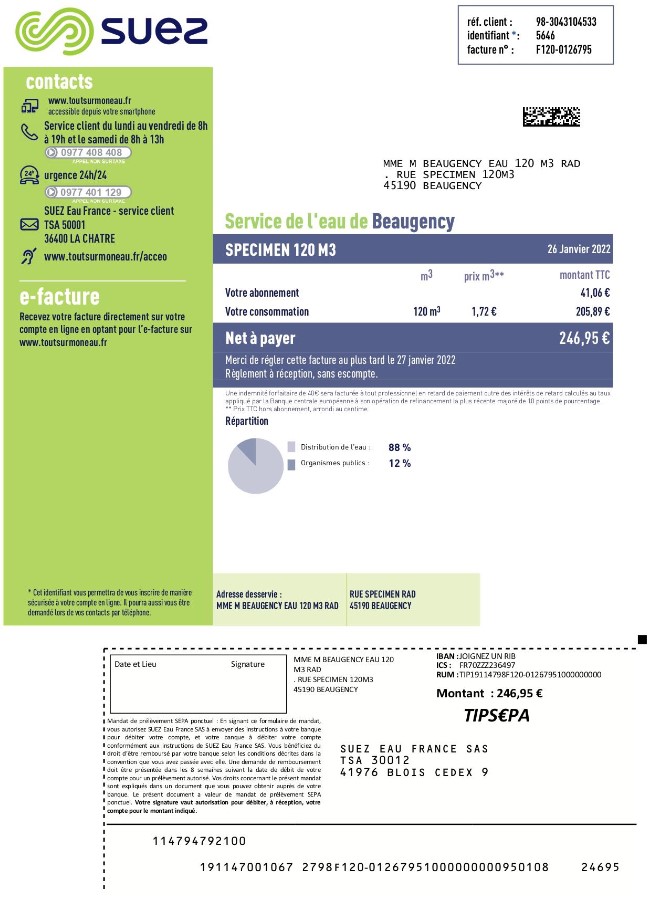
Extracted information |
---|
- Nom et prénom - Adresse - Adresse du justificatif - Nom et prénom du justificatif - Date du justificatif |
Gas bill
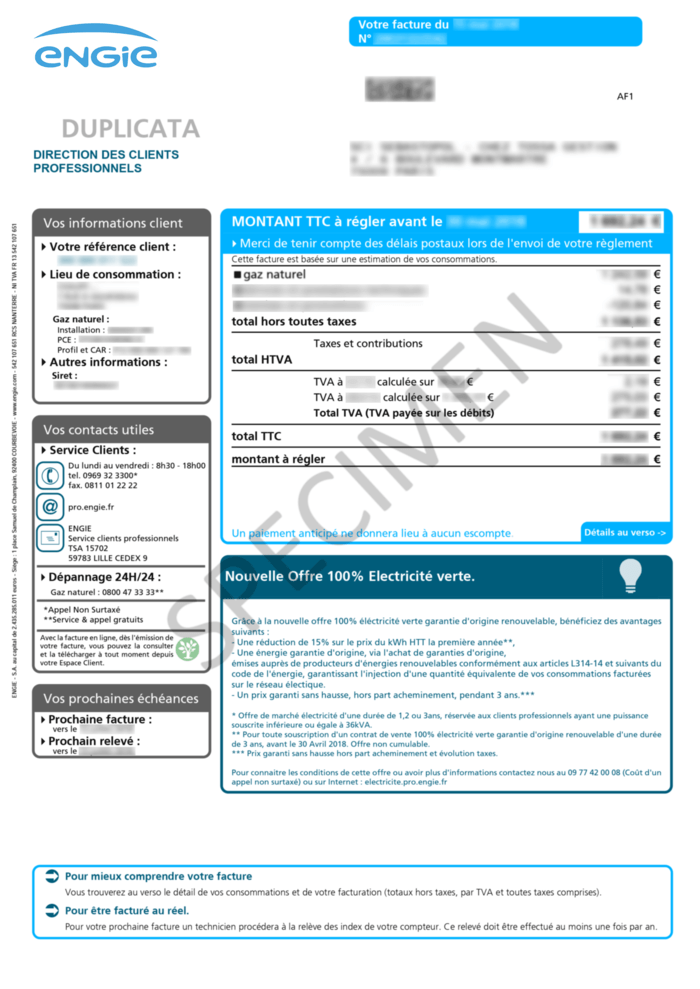
Extracted information |
---|
- Nom et prénom - Adresse - Adresse du justificatif - Nom et prénom du justificatif - Date du justificatif |
Energy bill
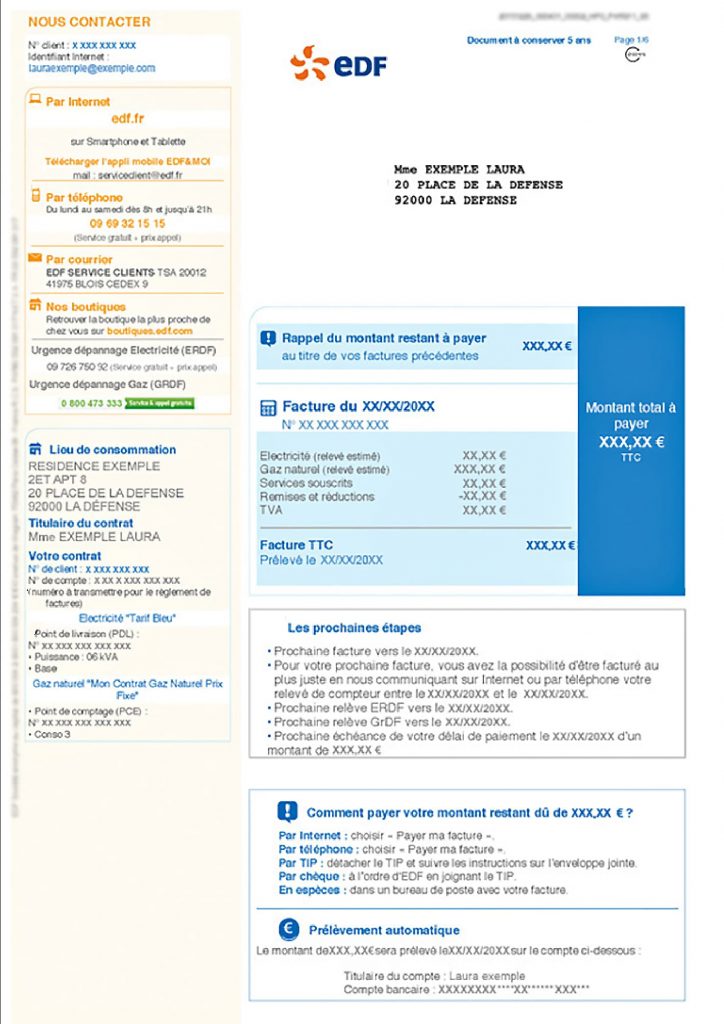
Extracted information |
---|
- Nom et prénom - Adresse - Adresse du justificatif - Nom et prénom du justificatif - Date du justificatif |
Internet bill
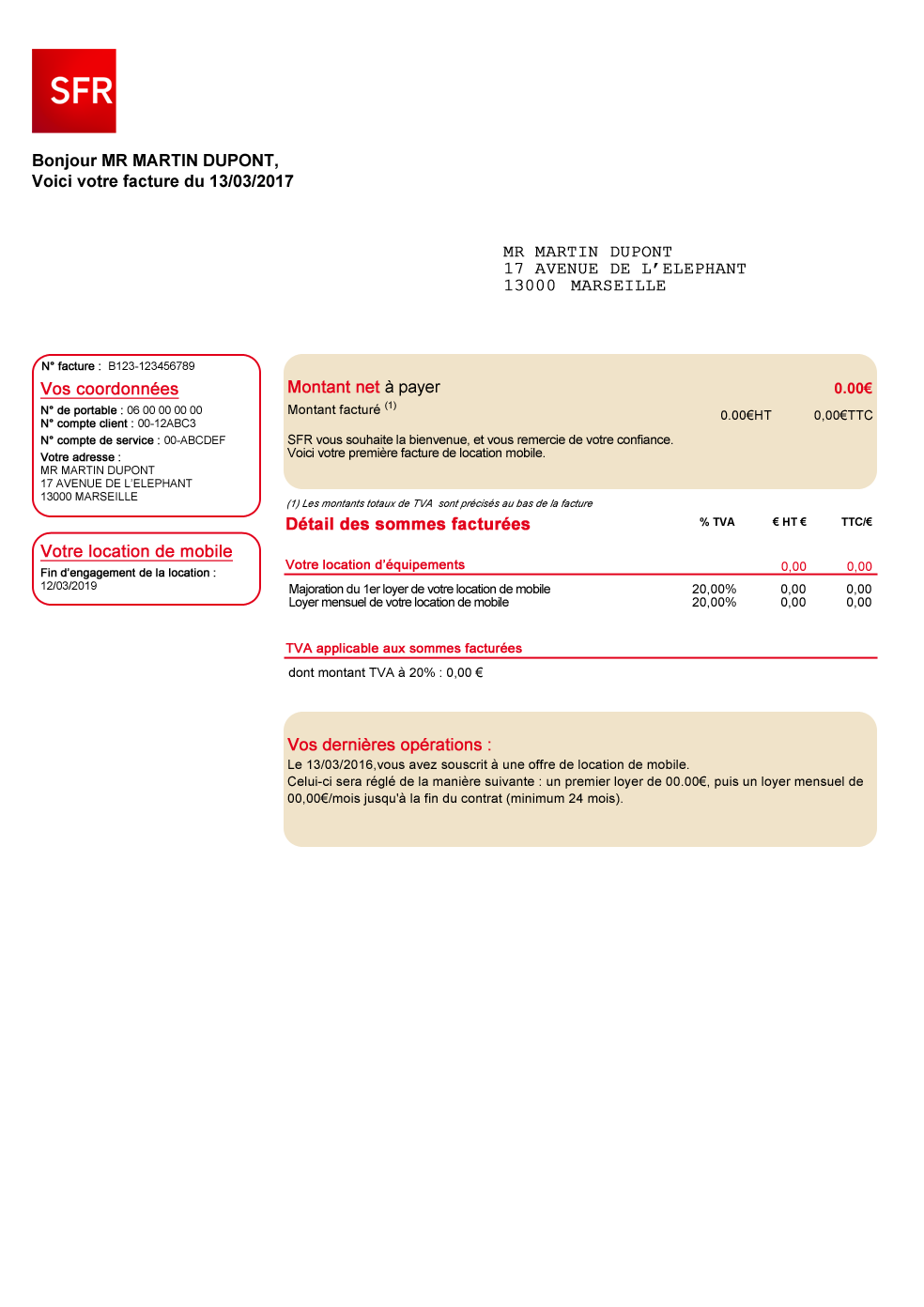
Extracted information |
---|
- Full name - Address of the owner - Address of the invoice - Full name on the invoice - Date of the invoice |
Setting up the API
The synchronous API model extracts data from submitted documents in real time.
API token is required
In order to perform any call, you will need an API token that can be retrieved thanks your API credentials. To learn about authentification, please refer to this page
curl --request POST \
--url https://api.datakeen.co/api/v1/reco/proof-of-address \
--header 'accept: application/json' \
--header 'content-type: application/json'
npm install api --save
const sdk = require('api')('@datakeen/v1.4.0#ax268r1ilnd0liqe');
sdk.postRecoProofOfAddress()
.then(({ data }) => console.log(data))
.catch(err => console.error(err));
require 'uri'
require 'net/http'
url = URI("https://api.datakeen.co/api/v1/reco/proof-of-address")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
response = http.request(request)
puts response.read_body
composer require guzzlehttp/guzzle
<?php
require_once('vendor/autoload.php');
$client = new \GuzzleHttp\Client();
$response = $client->request('POST', 'https://api.datakeen.co/api/v1/reco/proof-of-address', [
'headers' => [
'accept' => 'application/json',
'content-type' => 'application/json',
],
]);
echo $response->getBody();
python -m pip install requests
import requests
url = "https://api.datakeen.co/api/v1/reco/proof-of-address"
headers = {
"accept": "application/json",
"content-type": "application/json"
}
response = requests.post(url, headers=headers)
print(response.text)
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://api.datakeen.co/api/v1/reco/proof-of-address");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
CURLcode ret = curl_easy_perform(hnd);
dotnet add package RestSharp
using RestSharp;
var options = new RestClientOptions("https://api.datakeen.co/api/v1/reco/proof-of-address");
var client = new RestClient(options);
var request = new RestRequest("");
request.AddHeader("accept", "application/json");
request.AddHeader("content-type", "application/json");
var response = await client.PostAsync(request);
Console.WriteLine("{0}", response.Content);
CURL *hnd = curl_easy_init();
curl_easy_setopt(hnd, CURLOPT_CUSTOMREQUEST, "POST");
curl_easy_setopt(hnd, CURLOPT_WRITEDATA, stdout);
curl_easy_setopt(hnd, CURLOPT_URL, "https://api.datakeen.co/api/v1/reco/proof-of-address");
struct curl_slist *headers = NULL;
headers = curl_slist_append(headers, "accept: application/json");
headers = curl_slist_append(headers, "content-type: application/json");
curl_easy_setopt(hnd, CURLOPT_HTTPHEADER, headers);
CURLcode ret = curl_easy_perform(hnd);
(require '[clj-http.client :as client])
(client/post "https://api.datakeen.co/api/v1/reco/proof-of-address" {:content-type :json
:accept :json})
package main
import (
"fmt"
"net/http"
"io"
)
func main() {
url := "https://api.datakeen.co/api/v1/reco/proof-of-address"
req, _ := http.NewRequest("POST", url, nil)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := io.ReadAll(res.Body)
fmt.Println(string(body))
}
POST /api/v1/reco/proof-of-address HTTP/1.1
Accept: application/json
Content-Type: application/json
Host: api.datakeen.co
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url("https://api.datakeen.co/api/v1/reco/proof-of-address")
.post(null)
.addHeader("accept", "application/json")
.addHeader("content-type", "application/json")
.build();
Response response = client.newCall(request).execute();
const options = {
method: 'POST',
headers: {accept: 'application/json', 'content-type': 'application/json'}
};
fetch('https://api.datakeen.co/api/v1/reco/proof-of-address', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));
val client = OkHttpClient()
val request = Request.Builder()
.url("https://api.datakeen.co/api/v1/reco/proof-of-address")
.post(null)
.addHeader("accept", "application/json")
.addHeader("content-type", "application/json")
.build()
val response = client.newCall(request).execute()
#import <Foundation/Foundation.h>
NSDictionary *headers = @{ @"accept": @"application/json",
@"content-type": @"application/json" };
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:[NSURL URLWithString:@"https://api.datakeen.co/api/v1/reco/proof-of-address"]
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:10.0];
[request setHTTPMethod:@"POST"];
[request setAllHTTPHeaderFields:headers];
NSURLSession *session = [NSURLSession sharedSession];
NSURLSessionDataTask *dataTask = [session dataTaskWithRequest:request
completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (error) {
NSLog(@"%@", error);
} else {
NSHTTPURLResponse *httpResponse = (NSHTTPURLResponse *) response;
NSLog(@"%@", httpResponse);
}
}];
[dataTask resume];
opam install cohttp-lwt-unix cohttp-async
open Cohttp_lwt_unix
open Cohttp
open Lwt
let uri = Uri.of_string "https://api.datakeen.co/api/v1/reco/proof-of-address" in
let headers = Header.add_list (Header.init ()) [
("accept", "application/json");
("content-type", "application/json");
] in
Client.call ~headers `POST uri
>>= fun (res, body_stream) ->
(* Do stuff with the result *)
$headers=@{}
$headers.Add("accept", "application/json")
$headers.Add("content-type", "application/json")
$response = Invoke-WebRequest -Uri 'https://api.datakeen.co/api/v1/reco/proof-of-address' -Method POST -Headers $headers
library(httr)
url <- "https://api.datakeen.co/api/v1/reco/proof-of-address"
response <- VERB("POST", url, content_type("application/json"), accept("application/json"))
content(response, "text")
import Foundation
let headers = [
"accept": "application/json",
"content-type": "application/json"
]
let request = NSMutableURLRequest(url: NSURL(string: "https://api.datakeen.co/api/v1/reco/proof-of-address")! as URL,
cachePolicy: .useProtocolCachePolicy,
timeoutInterval: 10.0)
request.httpMethod = "POST"
request.allHTTPHeaderFields = headers
let session = URLSession.shared
let dataTask = session.dataTask(with: request as URLRequest, completionHandler: { (data, response, error) -> Void in
if (error != nil) {
print(error as Any)
} else {
let httpResponse = response as? HTTPURLResponse
print(httpResponse)
}
})
dataTask.resume()
Sending multiple documents
Scans, pictures and documents should be sent one by one. Front and back the same document cannot be sent in the same file. If you want to send multiple scans at the same time, please refer to the multi-docs API.
API Response
An instance of the usual response is displayed in the following JSON. You will find the complete information, including extraction and checks. More detailed examples of each extracted field are given below.
{
"extractedInformation": {
"JDDAddress": {
"confidence": 0.8124772316286862,
"value": "Bois et sens 1 rue de l arrondeau 49600 beaupreau en mauges"
},
"JDDName": {
"confidence": 0.7334919641685792,
"value": "PAUL DUPOND"
},
"invoiceDate": {
"confidence": 0.7962046397903759,
"value": "14/12/20"
},
"ownerAddress": {
"confidence": 0.8526500808687706,
"value": "Bois et sens 1 rue de i arrondeau 49600 beaupreau en mauges"
},
"ownerFullName": {
"confidence": 0.8164044355970418,
"value": "PAUL DUPOND"
}
},
"message": "",
"status": 200
}
Extracted information format
For each field, the confidence value indicates the degree of certainty of the extraction with regard to the data on the document.
Full name
The ownerFullName (key) of the contract ow is returned as a string (value).
ownerFullName | Value |
---|---|
value | string |
confidence | number |
"ownerFullName": {
"confidence": 0.8164044355970418,
"value": "PAUL DUPOND"
}
Address of the owner
The ownerAddress (key) of the contract owner is returned as a string (value).
ownerAddress | Value |
---|---|
value | string |
confidence | number |
"ownerAddress": {
"confidence": 0.8526500808687706,
"value": "Bois et sens 1 rue de i arrondeau 49600 beaupreau en mauges"
}
Address of the invoice
The JDDAddress (key) of the contract owner is returned as a string (value).
JDDAddress | Value |
---|---|
value | string |
confidence | number |
"JDDAddress": {
"confidence": 0.8124772316286862,
"value": "Bois et sens 1 rue de l arrondeau 49600 beaupreau en mauges"
}
Full name on the invoice
The JDDName (key) of the contract owner is returned as a string (value).
JDDName | Value |
---|---|
value | string |
confidence | number |
"JDDName": {
"confidence": 0.7334919641685792,
"value": "PAUL DUPOND"
}
Date of the invoice
The invoiceDate (key) of the contract owner is returned as a string (value).
invoiceDate | Value |
---|---|
value | string |
confidence | number |
"invoiceDate": {
"confidence": 0.7962046397903759,
"value": "14/12/20"
}
Additional information
Loaded scans must pass prerequisites
To provide a qualitative service and a comprehensive data capture, every picture, scan, or document sent to our API must comply with determined prerequisites which can be found on this page
API limitations
- Maximum size : 5 MB
- Maximum number of calls per minute : 10 calls
Updated 4 months ago
Ready to process proofs of address ? See our API Reference for detailed information